Five Different Ways to Create Objects in Java
A list of five ways to create objects in Java, how they interact with constructors, and an example of how to utilize all of these methods.
Join the DZone community and get the full member experience.
Join For Free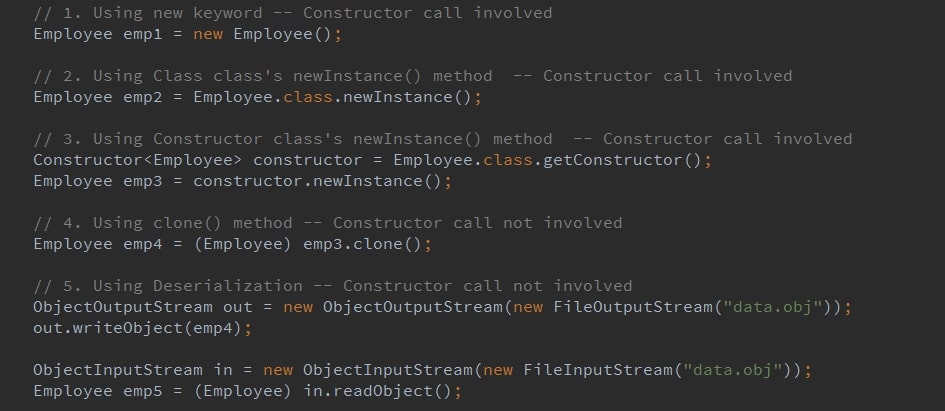
1. Using New Keywords
It is the most common and regular way to create an object and a very simple one also. Using this method, we can call whichever constructor we want to call (no-arg constructor and parameterized).
Employee emp1 = new Employee();
0: new #19 // class org/programming/mitra/exercises/Employee
3: dup
4: invokespecial #21 // Method org/programming/mitra/exercises/Employee."":()V
2. Using newInstance()
Method of Class Class
We can also use the newInstance()
method of a Class class to create an object. This newInstance()
method calls the no-arg constructor to create the object.
We can create an object by newInstance()
in the following way:
Employee emp2 = (Employee) Class.forName("org.programming.mitra.exercises.Employee").newInstance();
Or
Employee emp2 = Employee.class.newInstance();
51: invokevirtual #70 // Method java/lang/Class.newInstance:()Ljava/lang/Object;
3. Using newInstance()
Method of Constructor Class
Similar to the newInstance()
method of Class class, There is one newInstance()
method in the java.lang.reflect.Constructor class which we can use to create objects. We can also call the parameterized constructor and private constructor by using this newInstance()
method.
Constructor<Employee> constructor = Employee.class.getConstructor();
Employee emp3 = constructor.newInstance();
111: invokevirtual #80 // Method java/lang/reflect/Constructor.newInstance:([Ljava/lang/Object;)Ljava/lang/Object;
Both newInstance()
methods are known as reflective ways to create objects. In fact, newInstance()
method of the Class class internally uses newInstance()
method of the Constructor class. That's why the latter is preferred and used by different frameworks like Spring, Hibernate, Struts, etc.
4. Using clone()
Method
Whenever we call clone()
on any object, JVM creates a new object for us and copies all content of the previous object into it. Creating an object using the clone method does not invoke any constructor.
To use the clone()
method on an object, we need to implement Cloneable and define the clone()
method in it.
Employee emp4 = (Employee) emp3.clone();
162: invokevirtual #87 // Method org/programming/mitra/exercises/Employee.clone ()Ljava/lang/Object;
Java cloning is the most debatable topic in the Java community, and it does have its drawbacks, but it is still the most popular and easy way of creating a copy of any object until that object is full filling mandatory conditions of Java cloning. I have covered cloning in detail in a 3 article long, Java Cloning Series. Please go ahead and read them if you want to know more about cloning.
5. Using Deserialization
Whenever we serialize and then deserialize an object, JVM creates a separate object for us. In deserialization, JVM doesn’t use any constructor to create the object. To deserialize an object, we need to implement the Serializable interface in our class.
ObjectInputStream in = new ObjectInputStream(new FileInputStream("data.obj"));
Employee emp5 = (Employee) in.readObject();
261: invokevirtual #118 // Method java/io/ObjectInputStream.readObject:()Ljava/lang/Object;
As we can see in the above bytecodes, all four methods' calls get converted to invoke virtual (object creation is directly handled by these methods) except the first one, which got converted to two calls. One is new, and the other is invoke special (call to the constructor).
Example
Let’s consider an Employee class for which we are going to create the objects:
class Employee implements Cloneable, Serializable {
private static final long serialVersionUID = 1L;
private String name;
public Employee() { System.out.println("Employee Constructor Called..."); }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Employee employee = (Employee) o;
return Objects.equals(name, employee.name);
}
@Override
public int hashCode() { return Objects.hash(name); }
@Override
public String toString() { return String.format("Employee{name='%s'}", name); }
@Override
public Object clone() {
Object obj = null;
try {
obj = super.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return obj;
}
}
In the below Java program, we are going to create Employee
objects in all five ways.
public class ObjectCreation {
public static void main(String... args) throws Exception {
// 1. Using new keyword
Employee emp1 = new Employee();
emp1.setName("emp1");
// 2. Using Class class's newInstance() method
Employee emp2 = Employee.class.newInstance();
emp2.setName("emp2");
// 3. Using Constructor class's newInstance() method
Constructor<Employee> constructor = Employee.class.getConstructor();
Employee emp3 = constructor.newInstance();
emp3.setName("emp3");
// 4. Using clone() method
Employee emp4 = (Employee) emp3.clone();
emp4.setName("emp4");
// Serialization
try (ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("data.obj"))) {
out.writeObject(emp4);
}
// 5. Using Deserialization
Employee emp5;
try (ObjectInputStream in = new ObjectInputStream(new FileInputStream("data.obj"))) {
emp5 = (Employee) in.readObject();
emp5.setName("emp5");
}
System.out.println(emp1 + ", hashcode : " + emp1.hashCode());
System.out.println(emp2 + ", hashcode : " + emp2.hashCode());
System.out.println(emp3 + ", hashcode : " + emp3.hashCode());
System.out.println(emp4 + ", hashcode : " + emp4.hashCode());
System.out.println(emp5 + ", hashcode : " + emp5.hashCode());
}
}
This program will give the following output:
Employee Constructor Called...
Employee Constructor Called...
Employee Constructor Called...
Employee{name='emp1'}, hashcode : 3117192
Employee{name='emp2'}, hashcode : 3117193
Employee{name='emp3'}, hashcode : 3117194
Employee{name='emp4'}, hashcode : 3117195
Employee{name='emp5'}, hashcode : 3117196
You can find the complete source code for this article on this Github Repository, and please feel free to provide your valuable feedback.
Published at DZone with permission of Naresh Joshi, DZone MVB. See the original article here.
Opinions expressed by DZone contributors are their own.
Comments