Kotlin Coroutines and Delay
Whenever I suspect that there's a timing conflict causing a problem with rendering and directives, I usually opt for a JavaScript setTimeout
with a delay. The setTimeout
code never makes it to production, but it does help me to understand if my code is the problem or if there's a timing conflict.
In working with Kotlin on Android, I've needed to employ the same technique. Kotlin obviously doesn't have a setTimeout
, but it does have coroutines to achieve approximately the same effect.
To run an async coroutine with delay, you can use the following Kotlin code:
// Create an async coroutine
GlobalScope.launch {
delay(1000)
// Execute code to test functionality
}
The coroutine becomes async and the delay can be whatever amount of milliseconds you'd like!
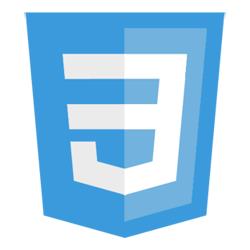
Feature detection via JavaScript is a client side best practice and for all the right reasons, but unfortunately that same functionality hasn't been available within CSS. What we end up doing is repeating the same properties multiple times with each browser prefix. Yuck. Another thing we...
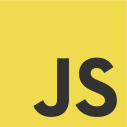
I remember the early days of JavaScript where you needed a simple function for just about everything because the browser vendors implemented features differently, and not just edge features, basic features, like addEventListener
and attachEvent
. Times have changed but there are still a few functions each developer should...
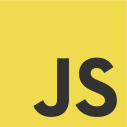
I was recently driven to create a MooTools plugin that would take an element and fade it to a min from a max for a given number of times. Here's the result of my Moo-foolery.
The MooTools JavaScript
Options of the class include:
min: (defaults to .5) the...
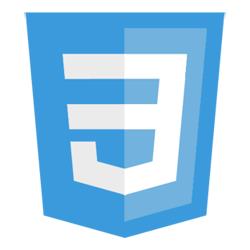
I'm a huge fan of WordPress' method of individual article deletion. You click the delete link, the menu item animates red, and the item disappears. Here's how to achieve that functionality with Dojo JavaScript.
The PHP - Content & Header
The following snippet goes at the...