Go go-to guide
yourbasic.org/golang
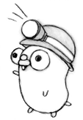
Why Go?
Step-by-step guides
- Go step by step
- Type, value and equality of interfaces
- Create, initialize and compare structs
- Slices/arrays explained: create, index, slice, iterate
- Maps explained: create, add, get, delete
- 5 basic for loop patterns
- 5 switch statement patterns
- Packages explained: declare, import, download, document
Go gotchas
- Assignment to entry in nil map
- Invalid memory address or nil pointer dereference
- Multiple-value in single-value context
- Array won’t change
- See all 27 gotchas
Tutorials
- Go tutorials
- Go beginner’s guide: top 4 resources to get you started
- How to use JSON with Go [best practices]
- Regexp tutorial and cheat sheet
- Java to Go in-depth tutorial
Cheat sheets
- Go string handling overview [cheat sheet]
- Conversions [complete list]
- fmt.Printf formatting tutorial and cheat sheet
- Format a time or date [complete guide]
- Regexp tutorial and cheat sheet
- Bitwise operators [cheat sheet]
- Start a new Go project [standard layout]
- Learn to love your compiler
Code for common tasks
- Go blueprints: code for common tasks
- 2 basic FIFO queue implementations
- 2 basic set implementations
- A basic stack (LIFO) data structure
- Access environment variables
- Access private fields with reflection
- Bitmasks, bitsets and flags
- Check if a number is prime
- Command-line arguments and flags
- Go as a scripting language: lightweight, safe and fast
- Compute absolute value of an int/float
- Compute max of two ints/floats
- 3 simple ways to create an error
- Create a new image
- Format byte size as kilobytes, megabytes, gigabytes, ...
- Generate all permutations
- Hash checksums: MD5, SHA-1, SHA-256
- Hello world HTTP server example
- How to best implement an iterator
- 4 iota enum examples
- Maximum value of an int
- Round float to 2 decimal places
- Round float to integer value
- The 3 ways to sort in Go
- Table-driven unit tests
Concurrent programming
- Concurrent programming
- Goroutines are lightweight threads
- Channels offer synchronized communication
- Select waits on a group of channels
- Data races explained
- How to detect data races
- How to debug deadlocks
- Waiting for goroutines
- Broadcast a signal on a channel
- How to kill a goroutine
- Timer and Ticker: events in the future
- Mutual exclusion lock (mutex)
- 3 rules for efficient parallel computation